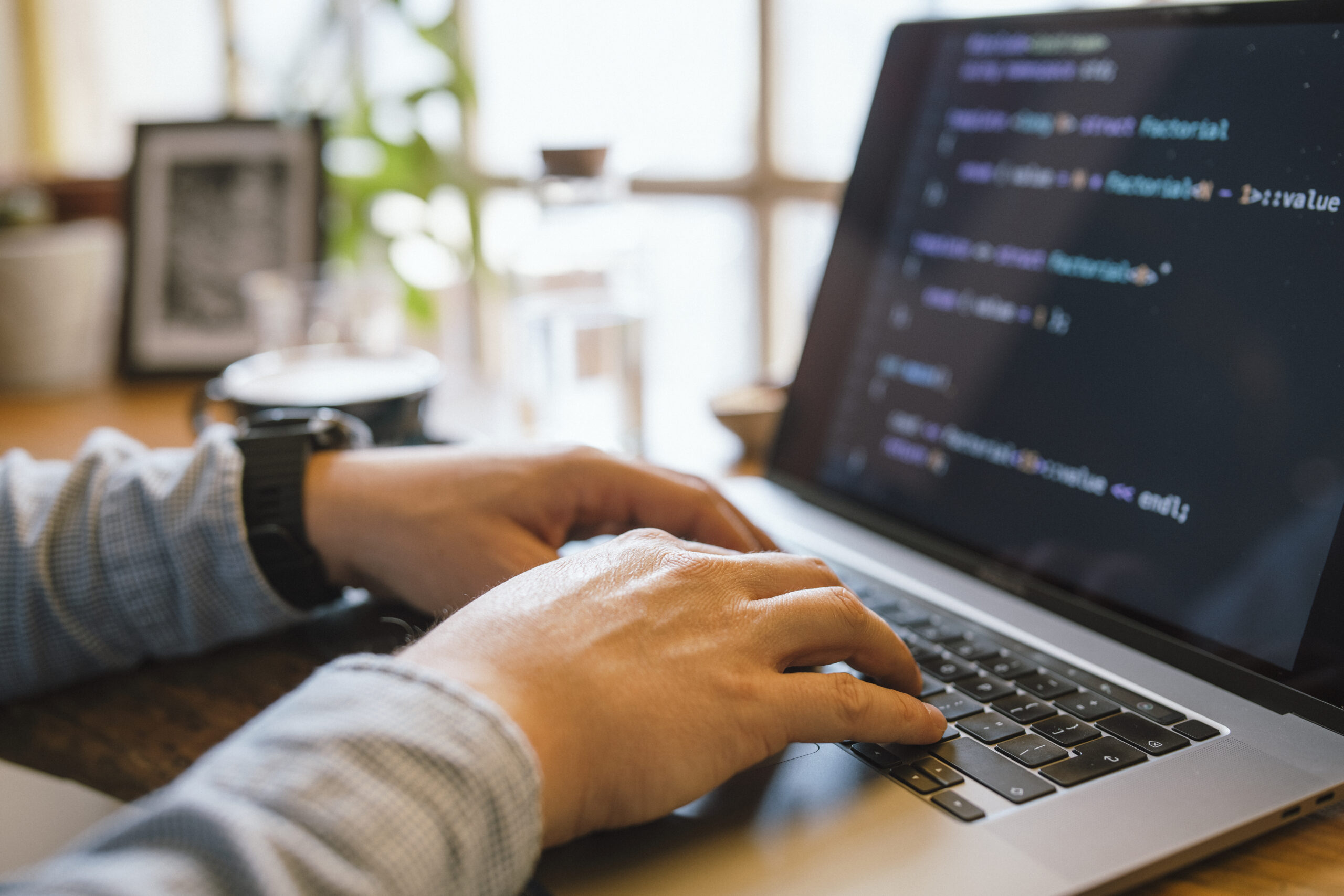
Debugging is one of the most vital — nonetheless often ignored — capabilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why points go Improper, and Understanding to Consider methodically to resolve troubles successfully. No matter if you're a beginner or a seasoned developer, sharpening your debugging skills can save several hours of irritation and drastically boost your productivity. Listed here are a number of methods to help developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest techniques developers can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though creating code is one particular Portion of improvement, knowing tips on how to communicate with it successfully during execution is equally significant. Present day improvement environments arrive Geared up with strong debugging abilities — but a lot of developers only scratch the surface of what these instruments can do.
Take, as an example, an Integrated Progress Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These resources allow you to set breakpoints, inspect the value of variables at runtime, action via code line by line, and even modify code over the fly. When utilised properly, they let you notice exactly how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer tools, which include Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, view actual-time performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can flip frustrating UI concerns into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging procedures and memory management. Studying these applications may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with Edition Handle programs like Git to be familiar with code history, discover the exact second bugs were being released, and isolate problematic changes.
In the end, mastering your resources implies heading over and above default configurations and shortcuts — it’s about acquiring an personal expertise in your development environment to ensure that when troubles occur, you’re not dropped in the dead of night. The higher you recognize your equipment, the more time you may shell out fixing the actual difficulty as opposed to fumbling via the process.
Reproduce the trouble
Just about the most vital — and often overlooked — ways in productive debugging is reproducing the situation. Right before leaping to the code or creating guesses, developers will need to make a steady setting or situation where the bug reliably seems. With no reproducibility, fixing a bug results in being a video game of prospect, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as possible. Check with queries like: What steps brought about the issue? Which natural environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the a lot easier it gets to isolate the exact disorders beneath which the bug takes place.
After you’ve gathered adequate information and facts, try and recreate the trouble in your neighborhood surroundings. This may imply inputting the exact same information, simulating very similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge cases or state transitions included. These checks not just enable expose the problem but in addition prevent regressions Later on.
From time to time, The difficulty might be setting-unique — it might transpire only on certain working programs, browsers, or underneath particular configurations. Utilizing equipment like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a step — it’s a attitude. It necessitates patience, observation, along with a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to fixing it. With a reproducible scenario, You should use your debugging resources a lot more efficiently, examination likely fixes properly, and converse additional Plainly with the staff or end users. It turns an abstract criticism right into a concrete problem — and that’s exactly where developers prosper.
Examine and Fully grasp the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when one thing goes Improper. Instead of seeing them as disheartening interruptions, developers should really study to deal with error messages as immediate communications through the program. They frequently let you know precisely what transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Begin by reading through the message thoroughly and in full. Quite a few developers, specially when beneath time strain, glance at the 1st line and right away begin making assumptions. But further inside the error stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Break the mistake down into components. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it place to a specific file and line number? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to know the terminology with the programming language or framework you’re utilizing. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and Discovering to recognize these can drastically quicken your debugging course of action.
Some errors are vague or generic, As well as in All those instances, it’s vital to look at the context during which the mistake happened. Check associated log entries, input values, and up to date variations within the codebase.
Don’t ignore compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more efficient and self-confident developer.
Use Logging Correctly
Logging is Among the most highly effective applications inside of a developer’s debugging toolkit. When used effectively, it offers real-time insights into how an application behaves, aiding you realize what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy begins with realizing what to log and at what degree. Typical logging levels include DEBUG, Facts, Alert, Mistake, and FATAL. Use DEBUG for thorough diagnostic details in the course of improvement, INFO for typical situations (like prosperous start off-ups), WARN for prospective problems that don’t crack the appliance, ERROR for actual complications, and Deadly when the procedure can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant information. Too much logging can obscure vital messages and slow down your system. Deal with essential functions, state variations, input/output values, and critical final decision points in the code.
Format your log messages Evidently and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Particularly precious in manufacturing environments where stepping by way of code isn’t possible.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a very well-thought-out logging technique, you could reduce the time it requires to identify challenges, acquire deeper visibility into your apps, and improve the Total maintainability and trustworthiness of your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To effectively determine and correct bugs, builders ought to approach the process like a detective solving a mystery. This frame of mind helps break down complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs of the challenge: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, accumulate just as much applicable information as you can without jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent picture of what’s going on.
Future, variety hypotheses. Check with on your own: What may very well be triggering this habits? Have any alterations not long ago been made into the codebase? Has this issue happened in advance of beneath equivalent circumstances? The goal would be to slender down alternatives and detect likely culprits.
Then, check your theories systematically. Attempt to recreate the condition in a very controlled environment. For those Gustavo Woltmann coding who suspect a certain function or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Pay back shut focus to small facts. Bugs frequently disguise while in the least predicted places—just like a lacking semicolon, an off-by-a person error, or simply a race problem. Be complete and individual, resisting the urge to patch The difficulty without having absolutely knowing it. Non permanent fixes could hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can save time for potential difficulties and assist Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and turn out to be simpler at uncovering concealed difficulties in complex techniques.
Publish Checks
Crafting tests is among the simplest methods to enhance your debugging capabilities and Over-all enhancement efficiency. Tests not just aid catch bugs early but in addition function a security Internet that gives you confidence when creating adjustments to the codebase. A properly-examined software is simpler to debug since it lets you pinpoint just the place and when a problem takes place.
Get started with device assessments, which center on particular person features or modules. These modest, isolated assessments can speedily reveal regardless of whether a selected bit of logic is Functioning as anticipated. Whenever a test fails, you immediately know where to glimpse, appreciably cutting down enough time invested debugging. Unit tests are Primarily practical for catching regression bugs—difficulties that reappear soon after Formerly being preset.
Upcoming, integrate integration tests and conclusion-to-conclude assessments into your workflow. These aid ensure that many areas of your application do the job collectively smoothly. They’re significantly valuable for catching bugs that happen in complex programs with a number of components or products and services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to Imagine critically regarding your code. To test a element correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code structure and much less bugs.
When debugging an issue, producing a failing test that reproduces the bug might be a robust first step. After the exam fails regularly, you may focus on repairing the bug and enjoy your test move when The difficulty is resolved. This strategy makes sure that the same bug doesn’t return Later on.
In a nutshell, producing tests turns debugging from a aggravating guessing video game right into a structured and predictable process—serving to you capture more bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the trouble—observing your display screen for several hours, seeking Remedy soon after Resolution. But One of the more underrated debugging applications is solely stepping absent. Having breaks allows you reset your intellect, reduce frustration, and often see the issue from the new perspective.
When you're as well close to the code for too long, cognitive fatigue sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your Mind gets significantly less effective at issue-solving. A brief stroll, a coffee break, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly all through extended debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Vitality and a clearer way of thinking. You could possibly all of a sudden detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
Should you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, however it essentially results in speedier and more effective debugging Eventually.
To put it briefly, using breaks will not be a sign of weak point—it’s a wise method. It presents your brain Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Just about every bug you encounter is more than just A brief setback—It can be an opportunity to develop being a developer. Irrespective of whether it’s a syntax mistake, a logic flaw, or perhaps a deep architectural concern, each can train you a little something valuable in the event you make time to reflect and analyze what went Incorrect.
Commence by asking by yourself some vital questions once the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The solutions typically reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see styles—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with all your friends could be Particularly powerful. No matter if it’s by way of a Slack concept, a short produce-up, or a quick knowledge-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary portions of your improvement journey. In fact, a number of the most effective developers are usually not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, each bug you correct provides a brand new layer on your skill set. So next time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a far more successful, confident, and capable developer. The following time you happen to be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.